How to structure a React application
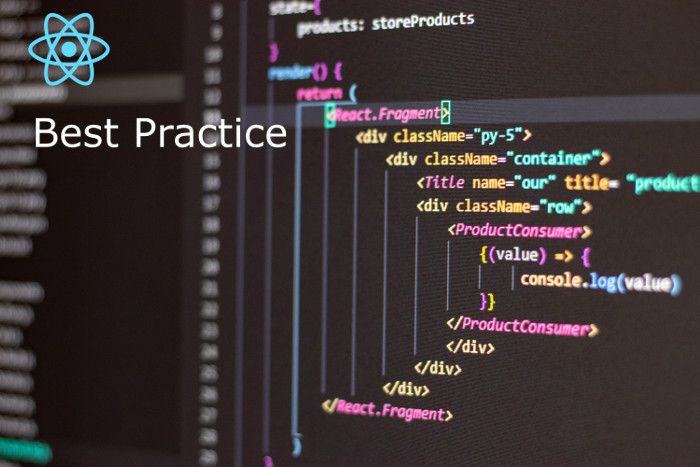
I have started ramping up my React skills and would like to share some best practices around structuring the project and how components should look like in a well-built React application.
-
A Component that manages the state should not be too involved with UI rendering. Its render method should be lean and should not contain too much jsx : This mostly applies to class-based components which are responsible for driving state in an application. Keeping this lean gives other developers along with you on the same application an idea of what components are involved in creating a particular section of the site.
-
Each component should have a clear focus: You will be tempted to add more than one functionality to a component, however, you should avoid overlapping purposes in one component to keep your application maintainable, not just by you but by other developers on your team as well.
-
Create granular components: Hierarchy is very important when creating components. Components should be nested as it gets granular to create a logical
-
Create as many as function components: This is very straight forward. Until the recent update of React, function components were not allowed to have a state. Even though it is now allowed in the latest React version. I highly recommend not to do that because imagine each component updating state and it will quickly get messy on where your state is being updated. Keeping it in a centralized place gives you more control over how the state is updated.
-
Have your container components as lean as possible (less jsx): These are high-level components that are responsible for gathering components in one place. This type of components should be lean as explained in #1
-
Most of your components should be presentational/stateless: This will help to manage the application by knowing in what direction the data is flowing. (From containers to components). This is in line with what I explained in #4
-
Use high-level components (HOC) when you want to apply specific logic globally: Here is an example on a HOC component that takes css classes with all the child components, wraps them into a div with classes applied to it.
Example implementation:
HOC component:
import React from 'React'; const WithClass = (WrappedComponent, className) => { return props => ( <div className={className}> <WrappedComponent {...props} /> </div> ); }; export default WithClass;
How to use HOC:
export default withClass(App, classes.App);
Usage: HOC components can be used for analytics events, handling errors etc. You can learn more about HOC at official React docs
Example structure: